WordPress is aggressively pushing for the implementation of theme settings via the WordPress Customizer. And it does make sense to have those design related settings in the Customizer. That’s because of the magical way the Customizer works. It reflects your settings real-time so that you can preview your changes before you accept them. Secondly, it does so without even having to save a setting into the database. Customizer achieves its functions via hooking into and filtering the user-submitted settings in the Customizer.
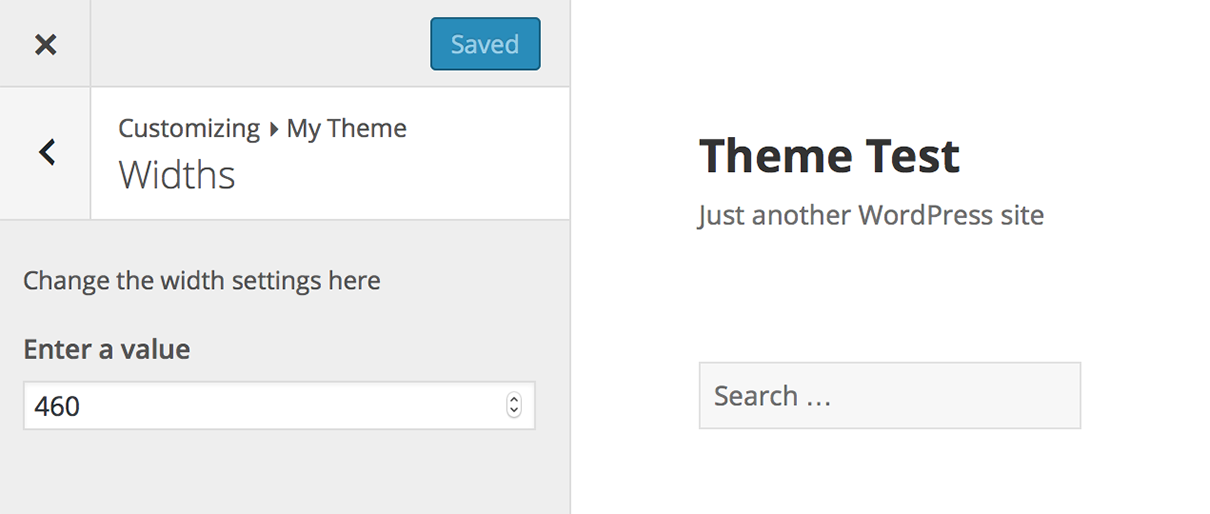
The best way to learn something is to get a piece of code that actually works and then tinker with it to your satisfaction. You learn a lot this way. On the other side, if you build something from scratch you’ll lose hours to get it to work, may even get frustrated and that ruins the learning process.
Here’s a working sample code that you can just copy paste and get started. The comments will help you in understanding what each line of code is doing.
// Add my settings into the customizer
add_action( 'customize_register', 'my_customizer_register' );
function my_customizer_register( $wp_customize ) {
$defaults = my_customizer_defaults();
$settings_type = 'theme_mod'; // What kind of setting? 'theme_mod' or 'option'
$transport = 'refresh'; // How does the preview/refresh take effect. Use 'postMessage' if you want to handle via js.
$wp_customize->add_panel( 'my_customizer_panel', array( // Add our top-level customizer panel
'priority' => 10, // Priority
'capability' => 'edit_theme_options', // Who can access this? Those who can edit theme options
'title' => __( 'My Theme', 'mytheme-td' ), // Title of this panel
'description' => __( 'Tune the raw power of My Theme', 'mytheme-td' ) // Description of this panel
) );
$wp_customize->add_section( 'my_customizer_val_section', array( // Add a section to (inside) our panel
'title' => __( 'Widths', 'mytheme-td' ), // Title of this section
'priority' => 30, // Priority, helps ot insert before or after other sections when you have multiple sections
'description' => __( 'Change the width settings here', 'mytheme-td' ), // Desctiption of this section
'panel' => 'my_customizer_panel' // Inside which panel to insert?
) );
$wp_customize->add_setting( 'mysetting_val', array( // Name of the setting
'default' => $defaults[ 'mysetting_val' ], // Default value of this setting
'type' => $settings_type, // Where will the setting be saves? As a theme_mod or option
'transport' => $transport, // How will the preview refresh? Use 'postMessage' if you want to handle via js.
'sanitize_callback' => 'absint' // How to validate this user submitted value? Insert your function name. absint is built into WP
) );
$wp_customize->add_control( 'mysetting_val_control', array( // Insert a customizer control (form-field) where the user can input the value
'label' => __( 'Enter a value', 'mytheme-td' ), // A descriptive label for this field
'section' => 'my_customizer_val_section', // The name of the section where this control is to be inserted.
'settings' => 'mysetting_val', // Which setting will this 'Control' control?
'type' => 'number' // What type of control is this? Try number, color, text etc. There are several built-in
) );
}
//My customizer's defaults setting(s). Insert other elements if you like
function my_customizer_defaults( ) {
$defaults = array( );
$defaults[ 'mysetting_val' ] = '460';
return $defaults;
}
// Make the settings do something. For now we can hook into the site header and out put some CSS
add_action( 'wp_head', 'my_setting_effect' );
function my_setting_effect( ) {
$width = get_theme_mod( 'mysetting_val' ); // Get the saved value. If it is missing, it will use the default value provided above
echo '<style type="text/css">#someelement {width:' . $width . 'px;}</style>'; // Output the CSS. Modify this to your requirements to make it have some effect.
}
The above code should work out of the box provided you fix the CSS (the very last line in the above code) to actually do something. I wouldn’t know which element you’d like to control so change the selector to your choice.
You can extend the above code one line at a time to insert any number of panels, sections, settings and controls for your purpose. The best way to use this is to put it into your theme’s functions.php or your plugin. I strongly recommend the WP Designer plugin that is meant specifically for these tasks.